Create an HTML landing page with Sass
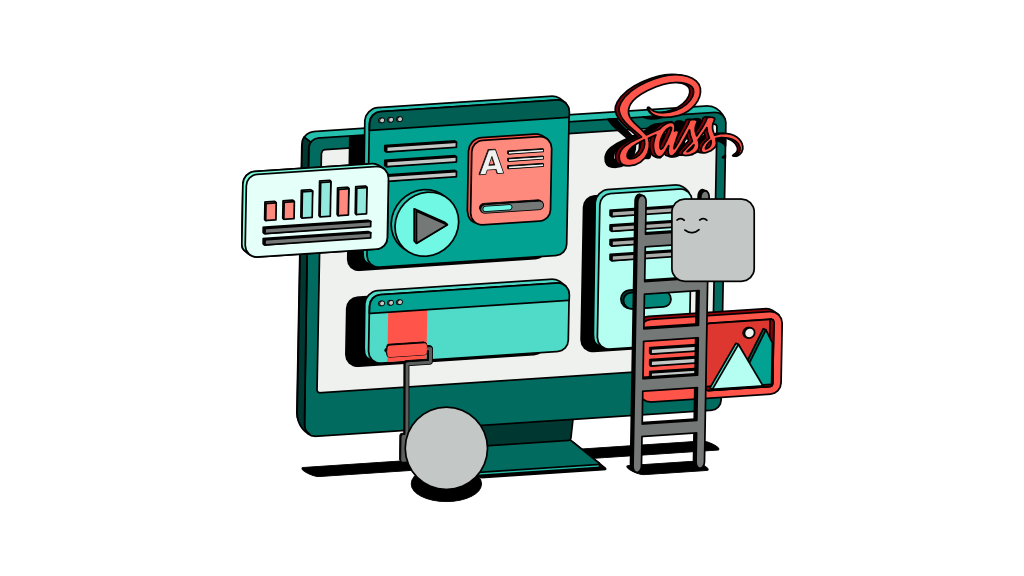
This is a simple tutorial to create a simple landing page using HTML and Sass only. As an experienced engineer, creating an HTML page can prove to be challenging as the tools you have in your possession can be an overkill to achieving a simple objective, such as building a landing page. In this tutorial, we’ll have a look at how to simplify this process and take the complexities out of the way with Sass.
Stripping down to the basics
For any landing page, all that you need is an HTML index page along with a CSS file to style it. You may potentially have some images and logos to display but we’ll get to that later.
Creating the HTML part of the landing page should be fairly straightforward and is the least of your worries. We all know how to create an HTML body tag and add a few paragraphs, incorporating a few <div> tags. But what about the styling part?
Plain CSS can be cumbersome due to the restrictive nature of its syntax. For more freedom, experienced software engineers most likely turn to Sass as it provides more flexibility to write CSS styles, with the ability to reuse certain components. However, Sass often comes pre-packaged within larger frameworks such as Ruby on Rails or Hapi.Js.
Using Sass on its own may not be a thought that comes to mind instantly, or perhaps the lack of experience in using Sass independently from other tools may be inhibiting.
In this tutorial we’ll go back to the basics, creating a plain and simple HTML page alongside a complex Sass structure that can be compiled into a single CSS file, all of which can be served directly through NGINX or any other web server of your choosing, without the complexity of a large framework codebase.
Steps
Install compass
For Sass to work, we will need a tool called ‘compass’ which can watch and compile our Sass code into plain CSS.
compass is written in Ruby, hence you need to install Ruby prior to installing compass.
If you don’t have it installed on your machine, you can use the following commands to install Ruby through your terminal.
$ gem update --system
$ gem install compass
Once you have compass installed, you can initialise the project as follows:
First, initialise your project. To do that, simply create a folder in your home directly. I prefer to do so using a terminal.
$ mkdir simpleProject
$ cd simpleProject
$ compass create
OUTPUT:
directory sass/
directory stylesheets/
create config.rb
create sass/screen.scss
create sass/print.scss
create sass/ie.scss
write stylesheets/ie.css
write stylesheets/print.css
write stylesheets/screen.css
Notice how compass has created a few files and folders - a readme.md file, a config.rb file, stylesheets and sass folders. We’re mainly concerned with the content of the file ‘config.rb’ which looks something like the following:
require 'compass/import-once/activate'
http_path = "/"
css_dir = "stylesheets"
sass_dir = "sass"
images_dir = "images"
javascripts_dir = "javascripts"
The file content is somewhat self-explanatory. It contains the sass directory ‘sass_dir’ path which compass is going to look into for .sass files, after which it will compile the .sass files into .css files, and store the output files into the 'stylesheets' directory.
I'm happy with the default configurations so I will leave it at that. Feel free to modify the folder structure as you wish.
Both the sass and stylesheets folders contain a few default files to start with but I won’t be using any of those so go ahead and delete all of it.
$ rm -rf sass/* stylesheets/*
Let’s get some basic HTML up
Now we have the basic project folder structure, so let’s add the final piece of the puzzle, the index.html page.
$ touch index.html
Once you have created the index.html file, you may paste the basic HTML structure below into your editor of choice after opening the file.
<!DOCTYPE html>
<html lang="en-US">
<head>
<title>Simple Project</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
</head>
<body>
<h1>Simple Project</h1>
</body>
</html>
Create the Sass folder structure
HTML, by default, will not accept a .sass or .scss files as it expects a .css file to be referenced as a stylesheet. Hence, we will need to create a main CSS file and reference it in the index.html file.
$ touch sass/styles.scss
Let’s test this by compiling the file just to make sure it yields the desired file.
$ compass compile
Notice how I use .scss instead of .sass. I like using the bracket-based syntax instead of indentations as they can get messy with different text editors. Feel free to use .sass instead.
The command above should yield the file stylesheets/styles.css. We now know the exact location of the plain 'vanilla' CSS file that can be imported into the index.html file.
Next, add the following line into your index file within the <head> block.
<link href="stylesheets/styles.css" rel="stylesheet" type="text/css" />
Great, everything is set and we’re now ready to start adding our HTML and CSS elements in their respective places.
Going back to our styles.scss file, it is good practice to let this file be a manifest file that contains import statements that reference other files containing the actual styling, while keeping this file free from hard-coded style elements.
Let’s start by creating a few basic directories within the sass directory.
$ mkdir sass/mixins sass/grid sass/layout sass/components sass/sections
We now have a basic sass project structure, let’s add some basic body style elements.
$ touch sass/layout/_body.scss
Notice how the file name starts with an underscore. This is to indicate to the sass compiler that this file is a fragment that is meant to be imported into a larger file, meaning it cannot be used alone and will not be compiled into a body.css file.
Next, add the following style elements into the newly created file.
body {
margin: 0;
padding: 0;
background-color: #eeeeee;
}
Going back to styles.scss, we need to add the following line to import the newly created file.
@import 'layout/body.scss';
Finally, we can go back to our terminal and compile the project
$ composs compile
You should see that the css elements are all stored in stylesheets/styles.css, a single file that can be imported into our HTML page. You can test the whole thing by simply opening your index.html file which should open up the browser page with a greyed out background and a header titled ‘Simple Project’.
What’s next
You can now start coding your landing page HTML elements and style them independently by taking advantage of Sass’ ability to import fragments, hence giving you the flexibility to create complex and reusable style elements, while remaining lightweight without the complexity of a full-fledged framework.
While you’re in an active development mode, you can ask compass to watch the folders specified in the config.rb file. It will then automatically detect changes to these files and compile them on the fly, giving the the ability to test your changes rapidly. To get compass to listen to changes, you can simply use the following command:
$ compass watch
This entire project can be compiled and checked into a git repository. You may choose not to check in your compiled code and instead compile it on the fly.
Remember to add .sass-cache into the .gitignore file. This folder contains temporary files generated by Sass while compiling which are not needed.
You can choose to add these project files into a lightweight NGINX container and serve those directly, or clone the repository onto a VM with NGINX installed to serve the static files.
This is, of course, not the only way to create a landing page, but it’s an easy and familiar way that is simple and lightweight enough. It serves the purpose of a landing page without consuming much computing power and resources.